GatsbyJS is a React framework and a Static Site Generator (SSG) tool used in building web applications. It combines Server Side Rendering (SSR) features, and static site development for building SEO-powered, secured, and fast applications.
In this article we’ll start with an introduction to Gatsby, we’ll learn the terms SSG, CSR, and SSR, how Gatsby improves SEO, and then we’ll build a simple website with Gatsby.
Introduction
GatsbyJS is built on React. React is a frontend UI library for frontend implementations. It supports the idea of small components that merges with other components to make bigger components.
As a UI library, React is a tool that can be combined with other tools for building web applications. Therefore, React on its own may require other tools (like routing tools, webpack server, and so on) for building full-fledged frontend applications.
With that being said, when you install React, you need to install other tools to make up your application. This results in an opinionated setup aided by Create React App (CRA). Despite this, more configurations and tools needed to be installed for a full application.
Then, Gatsby!
Gatsby is an opinionated framework that takes away the hassle of setting up the application and allows you to begin development immediately. Asides from this, Gatsby also solves the issue of Search Engine Optimisation (SEO) that using only React provides. react-helmet is not an effective SEO solution. This article explains that further.
SSR, CSR, and SSG
Client-Side Rendering (CSR)
In CSR, all routings and renderings are handled by the browser with JavaScript. For this technique, different HTML files are not created for different pages, instead, one page referencing some JavaScript files that determine what to display depending on the URL. React is a CSR tool. This means all routings are handled by the browser. In React, you have an index.html file found in the public folder which codes similar to this:
<html>
<head>
<title>React App</title>
</head>
<body>
<div id='root'></div>
</body>
</html>
After the build process (npm run build
), the index.html will look like this:
<html>
<head>
<title>React App</title>
</head>
<body>
<div id='root'></div>
...
<script src="/static/js/2.711c516a.chunk.js"></script>
<script src="/static/js/main.139dd313.chunk.js"></script>
</body>
</html>
The referenced .js
files handles all routings and responds to the URL with contents to share. build/index.html
is only fetched once, also with the JavaScript files. This may result in low page load speed due to fetching all resources. This method affects SEO in such a way that SEO crawlers only see <title>React App</title>
and does not see every other meta changes because those changes only happen when libraries like react-helmet
are executed (which is only on the browser).
Server Side Rendering
In contrast to CSR, SSR involves populating the browser with resources from the server. This means that for every route change, a request is made to the server to fetch new resources. SSR is perfect for SEO because SEO crawlers get the right meta information when any page is requested. SSR also has its cons, one of which is a delay when navigating between pages. CSR wins in this area because all JavaScript resources are fetched on the first request and every other navigation does not need a page refresh.
Static Site Generator
An SSG is a tool or set of tools that create static HTML pages from input files or content sources. Many SSG tools work in various ways but most of them take away the issues of security and slow fetching that database-driven platforms use. SSG takes content from different sources and builds them all into static pages which can be accessed faster when fetched by a browser.
How Gatsby improves SEO
Gatsby is an SSG tool that solves the issue of SEO that CSR brings and also makes routing faster compared to SSR. Gatsby does this by pre-building the web application before it is hosted. During the build process, all meta information provided within components is attached to the built pages. So when SEO crawlers or social sharing tools access any page of the application, they get access to the meta-information that has been provided to all pages during development. This does not involve any rendering in the browser. The built files are static pages which looks like each page was built separately like so:
<!-- /about -->
<html>
<head>
<title>About us</title>
</head>
</html>
Building a simple website with Gatsby
To show how Gatsby sites are built, we’ll be building a very simple website. No much complexities or dynamics, just simple.
Install the CLI tool
Firstly, install the gatsby
CLI tool. Or you can use npx
if that’s what you want.
npm i -g gatsby-cli
Create new site
You can either create a new Gatsby site with a basic template ([gatsby-starter-default]9https://www.gatsbyjs.com/starters/gatsbyjs/gatsby-starter-default/)) provided by the team, or use a specify another template to customize.
For the default template, a new site is created like so:
gatsby new new-site
Where new-site
is the name of the project you’re creating.
This gives the following project structure:
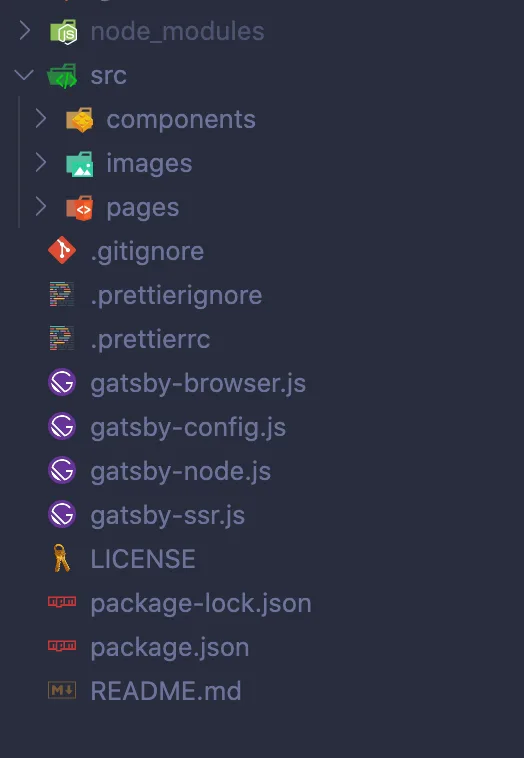
The template provides SEO configurations using GraphQL which you can improve. To see the site in action, run:
gatsby develop
At localhost:8000
, you’ll find your site displayed like so:
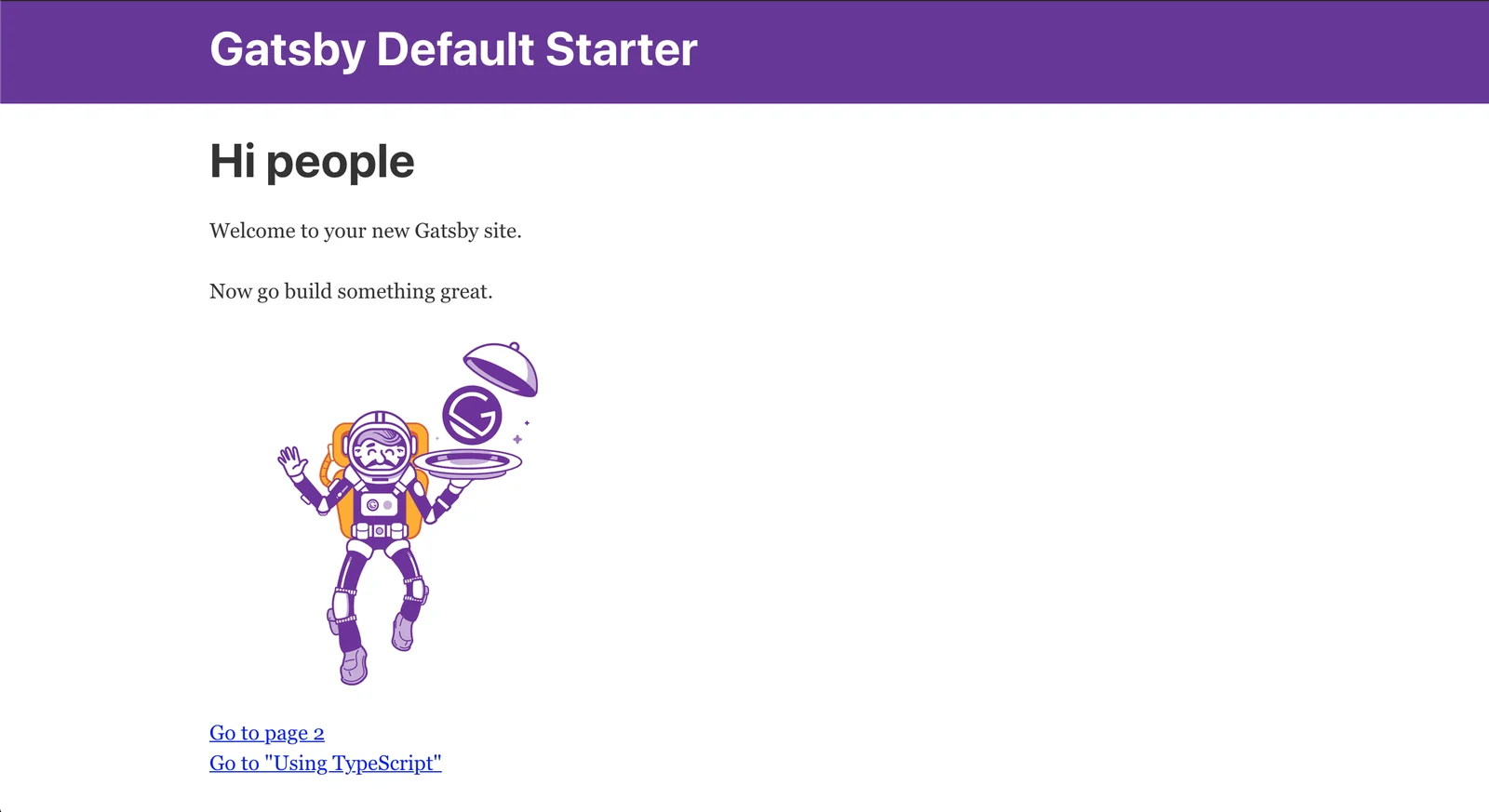
Alternatively, you can specify a template you want to use. You can find different starter templates from their list of starter libraries.
To use a template, say, gatsby-starter-blog, the following command will be used:
gatsby new my-gatsby-project https://github.com/gatsbyjs/gatsby-starter-blog
This gives the following project structure:
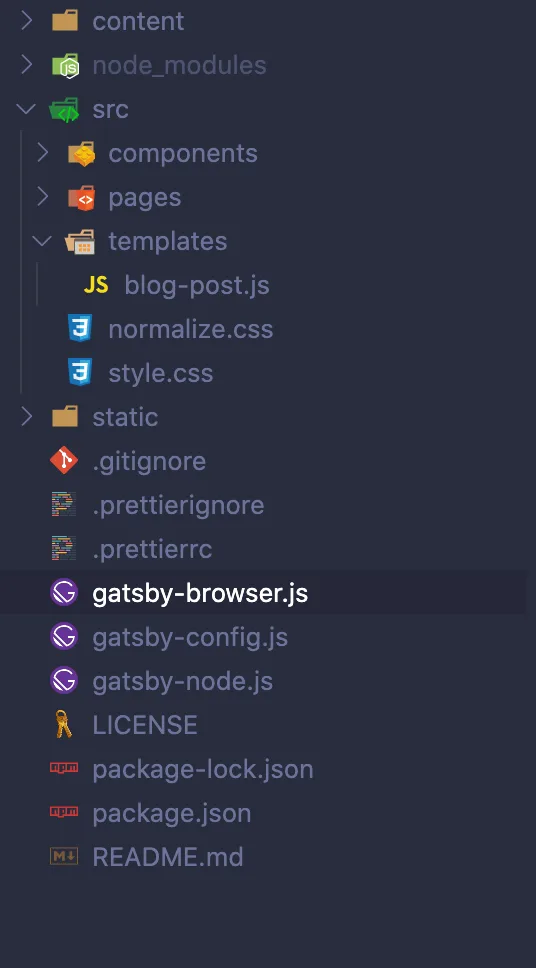
On starting the development server, localhost:8000
shows this:
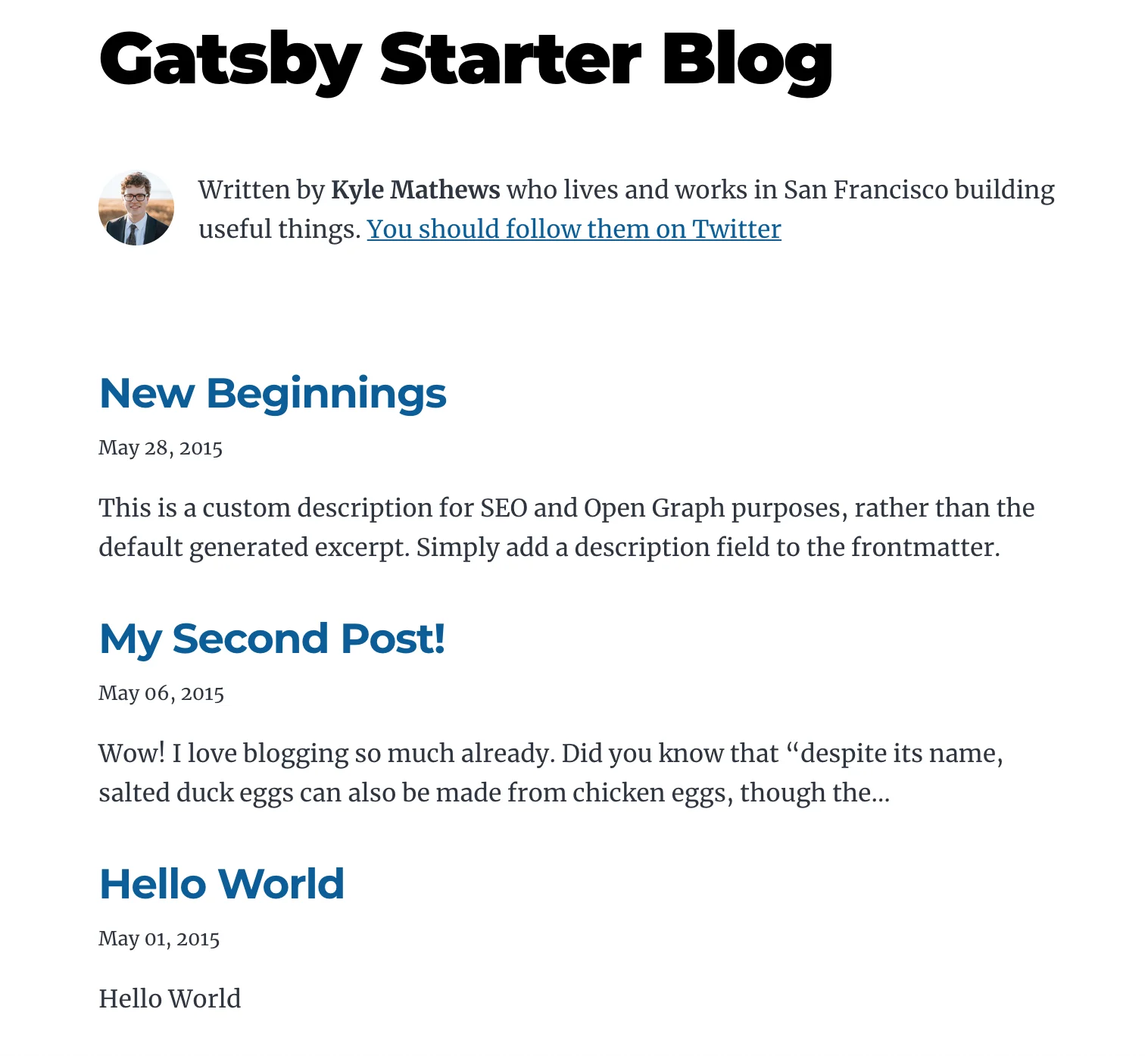
Improving the gatsby-starter-default template
The template has three folders under src
namely components, images and pages. The components and images page are optional, but pages is a required page for Gatsby. Unlike React, where you need a router library to show a set of components for a particular URL, in Gatsby, you create pages by having React JavaScript files under the pages folder.
Let’s add an about page under pages like so:
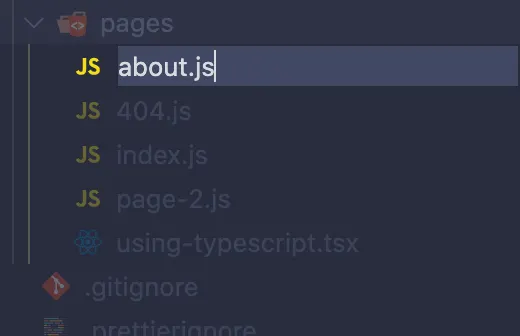
In about.js
, you can create your React components or import components. For example:
import React from "react"
import Layout from "../components/layout"
import SEO from "../components/seo"
const About = () => {
return (
<Layout>
<SEO title="About my website" />
<h1>About my beautiful website</h1>
</Layout>
)
}
export default About
SEO
is a component that dynamically updates meta information about each pages and Layout
is a wrapper component that serves as the layout of all pages. This can be configured to fit your needs too.
When you start your development server, go to localhost:8000/about
and you’ll find this:
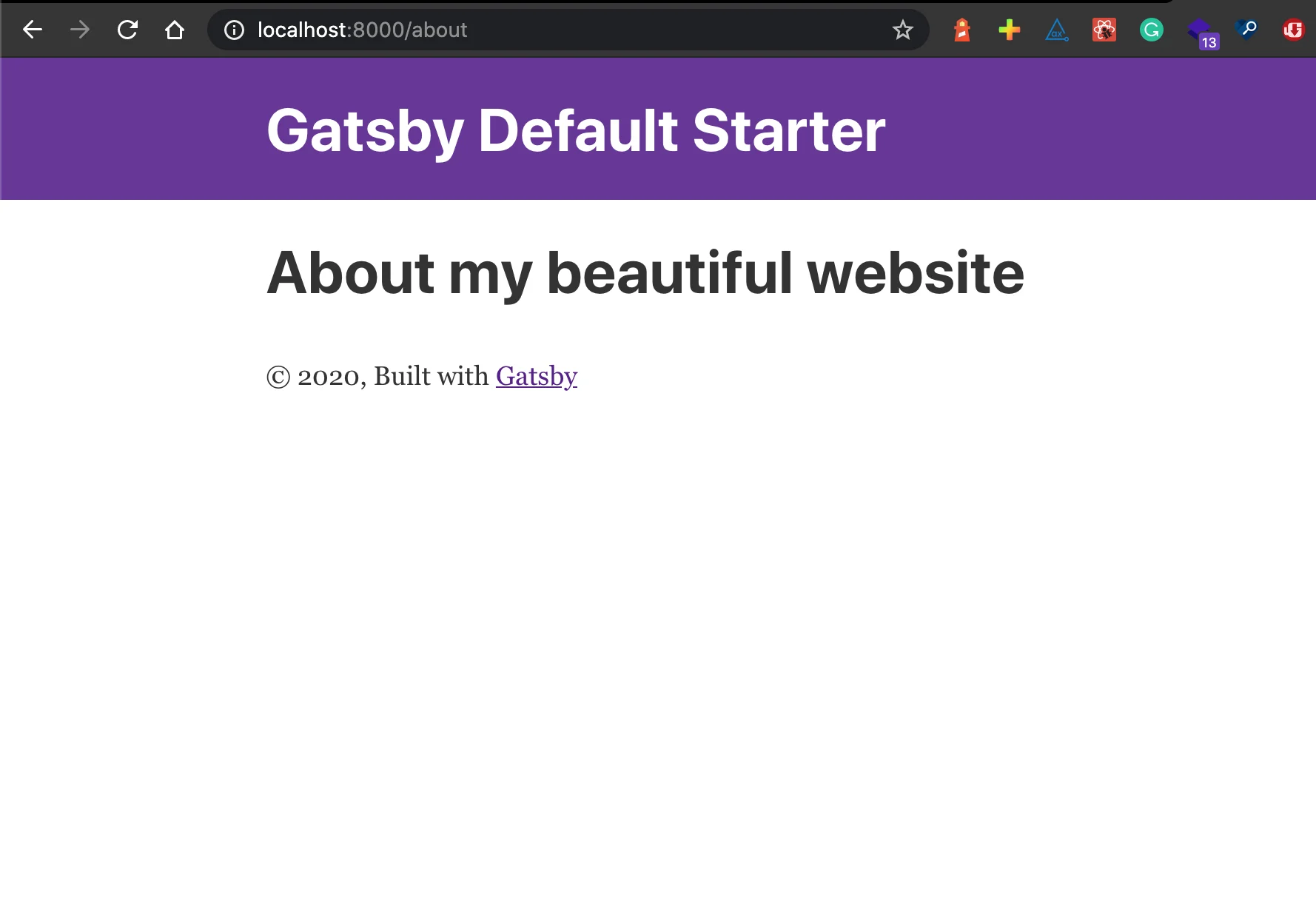
Note that: whatever you can do in React (components structuring, prop-types
, and so on), you can do the same in Gatsby. Gatsby makes things easier allow you to focus on the important parts of your application and pre-building your site to make SEO-fit. Also, Gatsby makes your site fast and since they are static pages, they can be served from anywhere (like CDNs).
Conclusion
Gatsby goes beyond the general understanding of “static pages”. Gatsby can source content from Content Management Systems and build static pages for them. An example is gatsby-source-instagram. It sources content from Gatsby at every building process, source the latest content from Instagram, and makes them available on your website.
There a lot of other awesome applications that can be achieved by using Gatsby, such as e-commerce tools, portfolios, and so on. Here's a gallery of sites using Gatsby.
Another beautiful thing about Gatsby is the community. You’ll find a ton of plugins that make development easier and more effective.
This article gives introductory information on what makes Gatsby an awesome tool. There are still more to learn to make the best use of Gatsby such as Gatsby and GraphQL, SSR APIs, and many more. Their documentation gives a very great guide to learning more about the tool.
I hope this article gives you reasons to try out Gatsby in your next project.