I'm so excited to see the recent release of Angular v11.0.0!
There are lot of improvements especially with regards to tooling.
What's new in the release
CLI: Inlining of Google Fonts
First, contentful paint is always the critical part for performance. [Eliminate render-blocking resources] (https://web.dev/render-blocking-resources/) is an important guide to improve the FCP performance, and Angular is also working on following the guide to inline the blocking resources.
In Angular v11, the Google Fonts will be inline by adding the following options to the angular.json
.
"optimization": {
"fonts": true
}
And without this option, your Google font importing looks like this.
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons">
After activating this optimization, it looks like this.
<style>
@font-face {
font-family: 'Material Icons';
font-style: normal;
font-weight: 400;
src: url(https://fonts.gstatic.com/s/materialicons/v55/flUhRq6tzZclQEJ-Vdg-IuiaDsNcIhQ8tQ.woff2) format('woff2');
}
.material-icons {
font-family: 'Material Icons';
font-weight: normal;
font-style: normal;
font-size: 24px;
line-height: 1;
letter-spacing: normal;
text-transform: none;
display: inline-block;
white-space: nowrap;
word-wrap: normal;
direction: ltr;
}
</style>
CLI: Hot Module Replacement Support
Angular supports HMR
for a while, but to make it work requires configuration and code changes, which is not a good developer experience. From v11, it is simple to enable the HMR
support by using
ng serve --hmr
Now, the live reload will also keep the state of your application, so it is much easier to apply some small updates to your application.
CLI: Experimental Webpack 5 support
Webpack 5 supports many new features such as:
- Module federation which is a new way to develop micro-frontend
- Disk caching for faster build
- Better tree shaking
Now, you can opt-in the Webpack 5 by adding the following section in the package.json file.
"resolutions": {
"webpack": "5.4.0"
}
CLI: ng build better output
Now, running ng build
will generate more clear output.
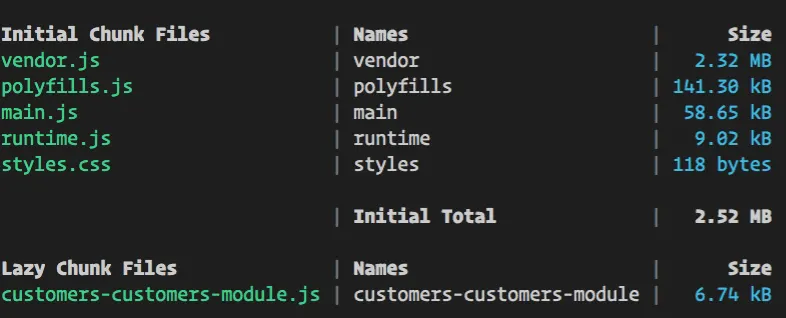
CLI: ng serve ask for new port
It is a really nice feature! If the 4200
port is in use, ng serve
will ask you for a different port without re-run with --port option.
? Port 4200 is already in use.
Would you like to use a different port? (Y/n)
** Angular Live Development Server is listening on localhost:60335, open your browser on http://localhost:60335/ **
CLI: Lint
Now, Angular uses TSLint as the default linter, and since TSLint is deprecated now, Angular uses angular-eslint
to replace TSLint/Codelyzer
. Here is the migration guide.
Features: Trusted Types support
Trusted Types is a new solution to prevent DOM-based cross-site scripting vulnerabilities.
Consider the following example:
anElement.innerHTML = location.href;
With Trusted Types enabled, the browser throws a TypeError and prevent this behavior. To tell the browser this is a trusted safe operation, you need to do it like this:
anElement.innerHTML = aTrustedHTML;
Note that the aTrustedHtml
is a TrustedHtml
object.
It looks similar to the DomSanitizer's functionality, so now Angular permits support to allow such an operation to return a Trusted Types.
Tooling
- Typescript is updated to v4.0
Deprecations
- IE 9,10 and IE mobile are no longer supported.
- ViewEncapsulation.Native has been removed.
async
test helper function is renamed towaitForAsync
.
For the full deprecation list, please check here.
Triaging issues and PRs
The Angular team put forward a significant effort to go through all the issues/PRs now opened in the GitHub, and check/triage these issues to make sure that they are at the correct state. It greatly helps the community to get better/faster support and feedback. I am so honored to also take part in this process.
How to update to v11
It is very simple. Just use the Angular CLI.
ng update @angular/cli @angular/core
You can find more information from update.angular.io/ and also from the official blog.