Many web applications utilize forms in places be it for logging in, making payments, or editing a user profile. As a user of web applications, you have probably noticed that the browser is able to autofill in certain fields when a form appears so that you don't have to do it yourself. If you've ever written an application in Lit though, you may have noticed that this doesn't always work as expected.
The Problem
I was working on a frontend project utilizing Lit and had to implement a login form. In essence these aren’t very complicated on the frontend side of life. You just need to define a form, put some input elements inside of it with the correct type attributes assigned to it, then you hook the form up to your backend, API, or whatever you need to call to authenticate by adding a submit handler.
However, there was an issue. The autocomplete doesn’t appear to be working as expected. Only the username field was being filled, but not the password. When this happened, I made sure to check documentation sites such as MDN and looked at examples. But I couldn’t find any differences between theirs and mine. At some point, I prepared a minimal reproducible example without Lit, and I was able to get the form working fine, so it had to do something with my usage of Lit.
After doing a little bit of research and some testing, I found out this happened because Lit relies very heavily on something known as the Shadow DOM. I don’t believe the Shadow DOM is necessarily supposed to break this functionality. But for most major browsers, it doesn’t play nice with autocomplete for the time being. I experienced slightly different behavior in all browsers, and the autocomplete even worked under Shadow DOM with Firefox in the Lit app I was working on.
The solution I ended up settling on was ensuring the form was contained inside of the Light DOM instead of the Shadow DOM, whilst also allowing the Shadow DOM to continue to be used in places where autofillable forms are not present. In this article I will show you how to implement this solution, and how to deal with any problems that might arise from it.
Shadow DOM vs. Light DOM
The Shadow DOM is a feature that provides a way to encapsulate your components and prevent unrelated code and components from affecting them in undesired ways. Specifically, it allows for a way to prevent outside CSS from affecting your components and vice versa by scoping them to a specific shadow root.
When it comes to the Light DOM, even if you’ve never heard of the term, you’ve probably used it. If you’ve ever worked on any website before, and interacted with the standard DOM tree, that is the Light DOM. The Light DOM, and any Shadow DOMs under it for that matter, can contain Shadow DOMs inside of them attached to elements. When you add a Lit component to a page, a shadow root will get attached to it that will contain its subelements, and prevent CSS from outside of that DOM from affecting it.
Using Light DOM with Certain Web Components
By default, Lit attaches a shadow root to all custom elements that extend from LitElement. However, web components don’t actually require a shadow root to function. We can do away with the shadow root by overriding the createRenderRoot method, and returning the web component itself:
createRenderRoot(): ShadowRoot | this {
return this;
}
Although we can just put this method in any element we want exposed into the Light DOM. We can also make a new component called LightElement that overrides this method that we can extend from instead of LitElement on our own components. This will be useful later when we tackle another problem.
Uh oh, where did my CSS styling and slots go?
The issue with not using a shadow root is Lit has no way to encapsulate your component stylesheets anymore. As a result, your light components will now inherit styles from the root that they are contained in. For example, if your components are directly in the body of the page, then they will inherit all global styles on the page. Similarly when your light components are inside of a shadow root, they will inherit any styles attached to that shadow root.
To resolve this issue, one could simply add style tags to the HTML template returned in the render()
method, and accept that other stylesheets in the same root could affect your components. You can use naming conventions such as BEM for your CSS classes to mitigate this for the most part. Although this does work and is a very pragmatic solution, this solution does pollute the DOM with multiple duplicate stylesheets if more than one instance of your component is added to the DOM.
Now, with the CSS problem solved, you can now have a functional Lit web component with form autofill for passwords and other autofillable data! You can view an example using this solution here.
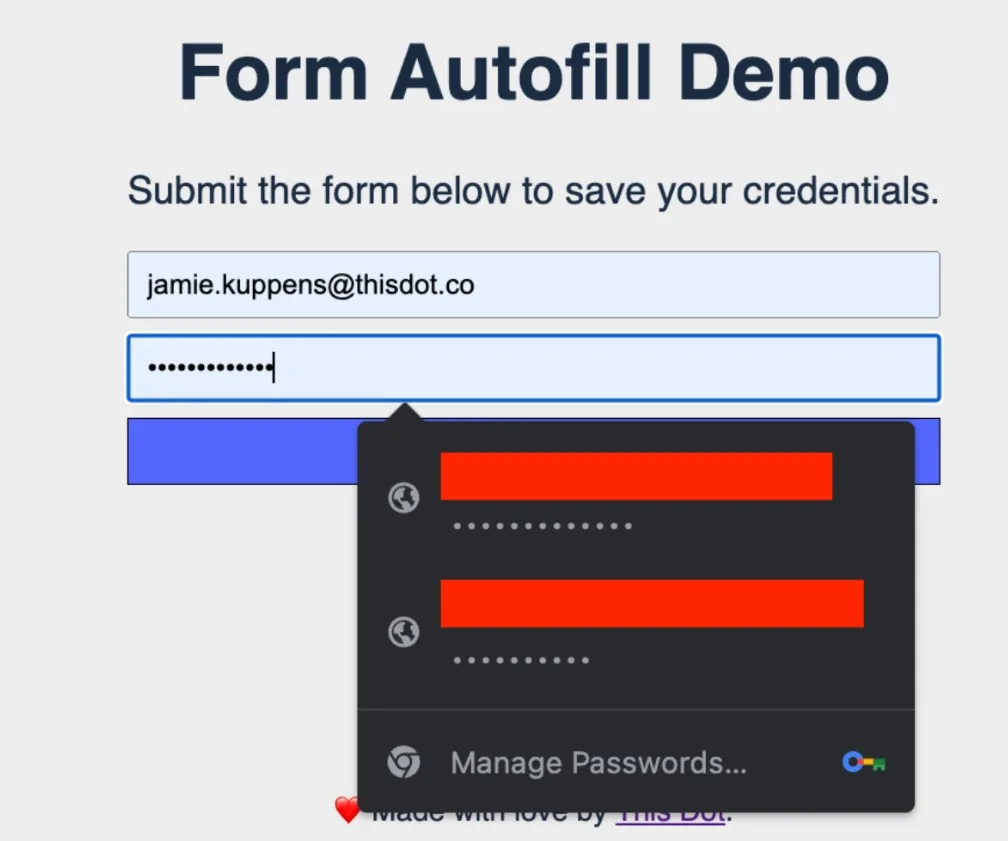
A Better Approach using Adopted Stylesheets
For a login page where only one instance of the component is in the DOM tree at any given point, the aforementioned solution is not a problem at all. However, this can become a problem if whatever element you need to use the Light DOM with is used in lots of places or repeated many times on a page. An example of this would be a custom input element in a table that contains hundreds of rows. This can potentially cause performance issues, and also pollute the CSS inspector in your devtools resulting in a suboptimal experience both for users and yourself.
The better, though still imperfect, way to work around this problem is to use the adopted stylesheets feature to attach stylesheets related to the web component to the root it is connected in, and reuse that same stylesheet across all instances of the node.
Below is a function that tracks stylesheets using an id and injects them in the root node of the passed in element. Do note that, with this approach, it is still possible for your component’s styles to leak to other components within the same root. And like I advised earlier, you will need to take that into consideration when writing your styles.
export function injectSharedStylesheet(
element: Element,
id: string,
content: string
) {
const root = element.getRootNode() as DocumentOrShadowRoot;
if (root.adoptedStyleSheets != null) {
evictDisconnectedRoots();
const rootNodes = documentStylesheets[id] ?? [];
if (rootNodes.find(value => value === root)) {
return;
}
let sharedStylesheet = sharedStylesheets[id];
if (sharedStylesheet == null) {
sharedStylesheet = new CSSStyleSheet();
sharedStylesheet.replaceSync(content);
sharedStylesheets[id] = sharedStylesheet;
}
root.adoptedStyleSheets.push(sharedStylesheet);
if (documentStylesheets[id] != null) {
documentStylesheets[id].push(root);
} else {
documentStylesheets[id] = [root];
}
} else {
// FALLBACK: Inject <style> manually into the document if adoptedStyleSheets
// is not supported.
const target = root === document ? document.head : root;
if (target?.querySelector(`#${id}`)) {
return;
}
const styleElement = document.createElement('style');
styleElement.id = id;
styleElement.appendChild(document.createTextNode(content));
target.appendChild(styleElement);
}
}
This solution works for most browsers, and a fallback is included for Safari as it doesn’t support adoptedStylesheets at the time of writing this article. For Safari we inject de-duplicated style elements at the root. This accomplishes the same result effectively.
Let’s go over the evictDisconnectedRoots function that was called inside of the injection function. We need to ensure we clean up global state since the injection function relies on it to keep duplication to a minimum. Our global state holds references to document nodes and shadow roots that may no longer exist in the DOM. We want these to get cleaned up so as to not leak memory. Thankfully, this is easy to iterate through and check because of the isConnected property on nodes.
function evictDisconnectedRoots() {
Object.entries(documentStylesheets).forEach(([id, roots]) => {
documentStylesheets[id] = roots.filter(root => root.isConnected);
});
}
Now we need to get our Lit component to use our new style injection function. This can be done by modifying our LightElement component, and having it iterate over its statically defined stylesheets and inject them. Since our injection function contains the de-duplication logic itself, we don’t need to concern ourselves with that here.
import { CSSResult, LitElement } from 'lit';
import { customElement } from 'lit/decorators.js';
import { injectSharedStylesheet } from './style-injector.js';
export interface SharedStylesheet {
id: string;
content: CSSResult;
}
@customElement('light-element')
export class LightElement extends LitElement {
static sharedStyles: SharedStylesheet[] = [];
connectedCallback() {
const { sharedStyles } = this.constructor as any;
if (sharedStyles) {
sharedStyles.forEach((stylesheet: SharedStylesheet) => {
injectSharedStylesheet(
this,
stylesheet.id,
stylesheet.content.toString()
);
});
}
super.connectedCallback();
}
createRenderRoot(): ShadowRoot | this {
return this;
}
}
With all that you should be able to get an autocompletable form just like the previous example. The full example using the adopted stylesheets approach can be found here.
Conclusion
I hope this article was helpful for helping you figure out how to implement autofillable forms in Lit. Both examples can be viewed in our blog demos repository. The example using basic style tags can be found here, and the one using adopted stylesheets can be found here.