Bun is a modern JavaScript runtime like Node or Deno focused on speed, and performance. It is an all-in-one tool (runtime, bundler, package manager, transpiler).
In this article, we will look at the excitement behind it, and dive into some features.
Overview
Bun is developed from scratch using the zig programming language. It uses JavaScriptCore Engine (Same with Safari browser), which is unlike Node.js and Deno, which use Chrome’s V8 engine. Bun natively implements hundreds of Node.js and Web APIs, including ~90% of Node-API functions (native modules), fs, path, buffer, and more. Plus, it supports Typescript and JSX out of the box.
Getting started
To install Bun on our machine, simply run the command:
curl https://bun.sh/install | bash
# Manually add the directory to your $HOME/.bashrc (or similar)
BUN_INSTALL="/home/jgranja/.bun"
PATH="$BUN_INSTALL/bin:$PATH"
For Mac, Linux, and Windows Subsystem.
Now run bun --version
to verify that it is correctly installed.
First Bun Script
Create a javascript file called http.js
and add the following:
export default {
port: 3000,
fetch(request) {
return new Response("Welcome to Bun!");
},
};
Now run the following:
bun run http.js
Then open http://localhost:3000 in your browser.
You can create the same file in Typescript as http.ts
and run:
bun run http.ts
Then open http://localhost:3000 in your browser.
Without modification or extra installation, we now have scripts in JavaScript and Typescript running.
Features
Let's dive into some of the features of bun.
Packages
Bun supports node packages and provides some integration with the latest React ecosystem with the create
command.
Bun uses node_modules.bun
to house all the imported dependencies. Let’s add React to our new project.
bun add react
Bun will generate the node_module.bun
file in the directory.
For an existing application similar to yarn
or npm
to install dependencies with bun, simply run bun install
in the project directory.
It will use the existing package.json
in combination with the lock file when present to add dependencies.
Scaffolding an App
To scaffold or create a new project from a template, framework (React), or blank project, use the create
command.
bun create blank ./blank-app
bun create bun-bakery ./bun-bakery-app
bun create discord-interactions ./discord-interactions-app
bun create hono ./hono-app
bun create next ./next-app
bun create react ./react-app
SQLite3 Out of the box
With Bun, you don’t have to install SQLite as it’s built-in out of the box.
import { Database } from "bun:sqlite";
// Create a new Database File
const db = new Database("db.sqlite3");
// Create a table in the database
db.run("CREATE TABLE IF NOT EXISTS people (name VARCHAR(100));")
// Insert Some Values into the table
db.run("INSERT INTO people VALUES ('hassan'), ('inidaname'), ('sani');")
// Query the table
const result = db.query("SELECT * FROM people;").all()
// Log results
console.log(result)
Run the code with bun run db.js
, and you should see the records that are inserted logged on the terminal.
Environment Variables
Bun automatically loads environment variables from .env
files. No more require("dotenv").config()
and you can simply access proccess.env
without needing to install packages.
Create an .env
file with the following:
APIKEY=NotReallyA_Key
Create a file http.js
export default {
port: 3000,
fetch(request) {
console.log(process.env.APIKEY)
return new Response("Welcome to Bun!");
},
};
Run the code with bun run http.js
, and you should see the NotReallyA_Key
logged on the terminal.
Conclusion
Hopefully this article showed you how easy it is to get started with Bun and the features you should be excited about.
The Bun approach to performance is truly a big win for the community.
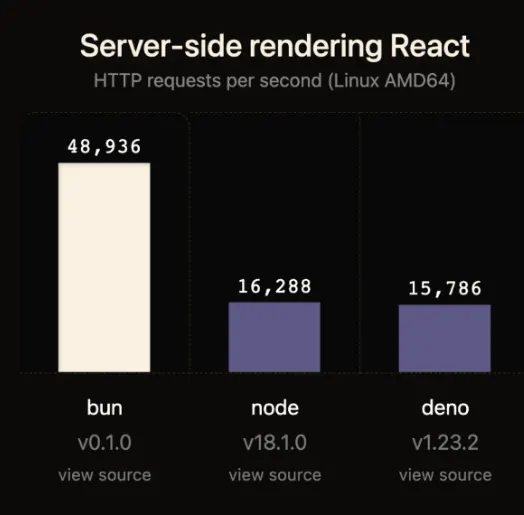
Bun is still young and seems to have a promising future. Will Bun replace Deno or Node? That is too early to call, as both existing runtimes have been around for a while and are actively maintained and features are being added often with constant improvements on the existing APIs.
Bun does not yet have a stable release (as of this writing) and is still very early in development.
What’s your opinion on bun or some exciting projects you might build or migrate with bun?